This is my third attempt at the “Idiot Level” Passport2Pain course, and my third completion (2013, 2016, and 2018). In 2016 I left my GPS at home and apparently didn’t bother to write anything up, so any comparisons will be to 2013.
My wife and I went over Friday afternoon to have dinner with and stay at the house of one of our ski instructor friends, who very conveniently lives 15 minutes from the starting line. I slept poorly as is my usual before big rides, but got up, skipped breakfast, and we headed over to the starting point.
After the usual wait and ride introduction (“In thinking about fundraisers, we had an idea. It wasn’t a *good* idea, but it was an idea…”), we queued up to start. They start with 4-5 riders every 30 seconds or slow to spread the riders out. Contrary to the pre-ride description, they made no effort to actually send out the idiot (80 mile) route riders first; I knew to line up near the front but I would have been upset if I had to wait 40 minutes to start. Considering the difference between the two routes is well over two hours, they need to do better at this.
We pedaled away from the start at Jensen Point, which is on this weird little spit. I started talking with a guy in a t shirt, jersey, and cutoffs; he had forgotten his clothes. He pulled ahead and took the first turn to exit the park area and immediately pancaked on this left side.
It had, you see, rained the night before and it was 57 degrees and cloudy. So there was still a bit of moisture on the road, and likely a bit of oily film.
He was fine and we rode off to start our quest, and I made a mental note not to ride too near to him – or any other riders – while the roads were wet.
I generally describe P2P as riding all the way around Vashon island and taking every road that goes down to the beach.

That is hyperbole. There are, in fact, numerous hills that will will not ride down, but we will ride down a large number; overall, there are 25 climbs on the ride, most of which are in the 200-300’ range, plus a bunch of small hills and rollers. If you are doing a ride like RAMROD, there are really only 3 hills (Paradise, Backbone ride, and Cayuse), and that’s how you track your progress. On P2P they do have checkpoints where you get your passport stamped, but there are 18 of them.
My approach is to just ride; I know what the parts are, and I know that I need to ride slow because the last set of hills is pretty bad.
So, we head off, do a short climb, and then descend down to the first real climb, which is a weird down and up. And quickly run into our first issues.
We roads and steep climbs do not mix. Going down you can just take it easy, and even with disc brakes I’m taking it easy on the still wet roads. The problem is when you start having to go up again. I can sit and ride up a 15% hill pretty easily and tough out a 20% hill, but it’s nice to be able to stand. Except if you do that, your rear wheel spins up. Which is bad. So, you just need to sit and suffer.
The first 5 stops go by pretty fast; slow and careful on the descent, and then doing my best on the climbs to stay smooth and keep calm. This part of the ride is the warmup, though it’s a little nuts that the warmup has 8 main climbs and 2500’ of up over the first 25 miles. We then have 5 miles with a climb or two, and then turn off onto Burma Road.
Burma is a mostly paved goatpath that rolls up down and around; they laid asphalt with doing as little grading as possible. Burma has one easy climb – say 13% or so – and then two hard climbs. They are aren’t very high, but they are well in excess of 20% (my GPS said 27 but I really don’t think they are quite that bad). The general way to attack Burma is to be able to ride slowly – say 3MPH – while standing, and if traffic permits, do a slight weave back and forth. It’s not categorically different than “The Widowmaker” in Sufferin’ Summits, and it’s quite a bit shorter, though it’s barely one lane wide.
That is what I did on two previous rides, but Burma is fully shaded and quite wet this year. After spinning the rear up despite being really gentle, I just ride slowly and muscle my way up. Not fun at all and I’m stressing my legs much more than I hoped, but it’s either that or just fall over (I don’t think I could unclip and stop), so I ride up both pitches apprehensively and then get to meet the devil.
Almost directly after, there’s another hill with a torn up descent at the bottom where you can barely stop and an ascent where you can’t stand, but that’s par for the course. Later this same hill has a solid 20% section, but luckily that pavement is dry and a real road so you can tack/paperboy back and forth and stand if you want to.
There’s one more loop down to the water, a spin along the main highway, and we hit the lunch stop.
I’ve been snacking a bit along the way; I have some nuts and I’m eating small amounts of carbs, and that’s working great except for 5 peanut M&Ms that give me a knot in the stomach.. At this point, I’m pretty tired and deciding whether to do the 55 mile or the 80. I eat the fillings of a very forgettable BLT and a bit of bread and then stop at the Thriftway for a Coke Zero but am stuck with a Diet Coke.
I mean, seriously, what are they thinking?
I text Kim to let her know where I am (she is doing a ride into the village for coffee) and tell her I’m 50/50 on which variant I will do and I’ll text her when I decide.
I roll out. There’s a small and ugly climb on the next section, but this one is dry and I’m feeling decent until my right hamstring starts to cramp near the top of the hill. I stop, dig some electrolyte capsules out of my pack, and wash them down. Then it’s off to Evil Twin #1 and #2. They really aren’t that bad and I’m climbing a bit better after food and Diet Coke. The second stop has chips and guac, and I have a few, heavy on the Guac.
And that’s all the hills on the 50 mile/6500’ route, so I need to make a decision. My toes and left shoulder hurt a little, but my legs are feeling okay, so I stop to text Kim and press on, onto Maury Island, and get ready to grit my teeth. Because as tough as the Burma Road section is, this section is a real bastard. It looks like it won’t be that bad – there are only 5 stops – but it’s a full 30 miles and over 3000’ of up.
We work our way through 14, and then descend down to 15. This has the added pain that as I near the stop, I ride by our friend’s house and out in front my Outback is parked, with a perfect bike-shaped space in the back, beckoning to me. I manage to avoid the temptation, but man, the hill out is a major bitch, and I’m tacking back and forth for all I am worth. And it’s not like I’m getting passed much, since all the fast people are in front those near me are bound together in a brotherhood and sisterhood of suffering and pain.
On the plus side, I’ve had no more cramping issues, so there is that.
Then there’s an ugly descent, and we ride into Dockton. We have three stops left, so three hills, right? How bad could that be?
I hate Dockton. We are down right at the water, but we climb 300’ up to the top of the island, and then we descend all the way to the water down yet another sketchy, wet, and slightly mossy road. For a measly stamp on our passports. Then we climb out that same damn hill, though the way from the water is worse.
And then – and this is the wonderfully terrible part of this ride – we do it again. Climb 200’ up into the hills, all the way down to the water, collect our penultimate stamp, and then it’s another 250’ climb back to where we started.
And the pain of Dockton is over. At this point I’m feeling pretty good; I *know* can finish the last hill, and then there are only a few rollers after that. I ride up a 150’ uncategorized hill – I mean really, it’s only a 7% and it feels very easy – in company with another rider, and I form a plan.
There is only one hill left and my legs feel like they have a little something left. So, we come to the last hill – 330’ of fun or so – and I start climbing hard, which is somewhere between 230 and 280 watts as the hill steepens and eases (my earlier target was <200 watts if I could). My data shows that I’m 43 seconds faster than my 2013 ascent – a full 9% faster. I get to the top, have a little bit of popcorn, and spin out to finish the ride.
The way back is about 4 miles with only a few rolling hills, so I push my speed up a bit. And then, finally, I finish, and get to have some well-earned barbecue with my spouse. It’s pretty good in the “Puget Sound Barbecue” category, but the brisket needed another couple hours in the smoker.
Analysis
Strava says I pulled 12 PRs on the route, and 9 of those were on hills. That makes me pretty happy, and I felt strong for most of the ride. It’s so much nicer and prettier than Sufferin’ summits.
Stats:
80.81 miles
7:11:46 riding time
9,949’ of up
11.2 mi/hr average
How does that compare to 2013? Well, in 2013 I rode 1.5 miles farther, which was probably due to more back-and-forth across the road, and finished in 7:13:27. My average speed then was 11.4 mil/hr, faster than this year, but my speed on descents was at least 25% slower than before because of the wetness.
2013’s ride was done on my Trek Madone, a fine bike for making speed but it was pretty harsh on the crappy Vashon roads. This year I was on my Roubaix with disc brakes, a frame tuned to soak up vibration, shocks in the seat and steering head, and 28mm tires at 80 psi. It was gloriously better; the stuff that really would have beat me up last year was still annoying but not too bad.
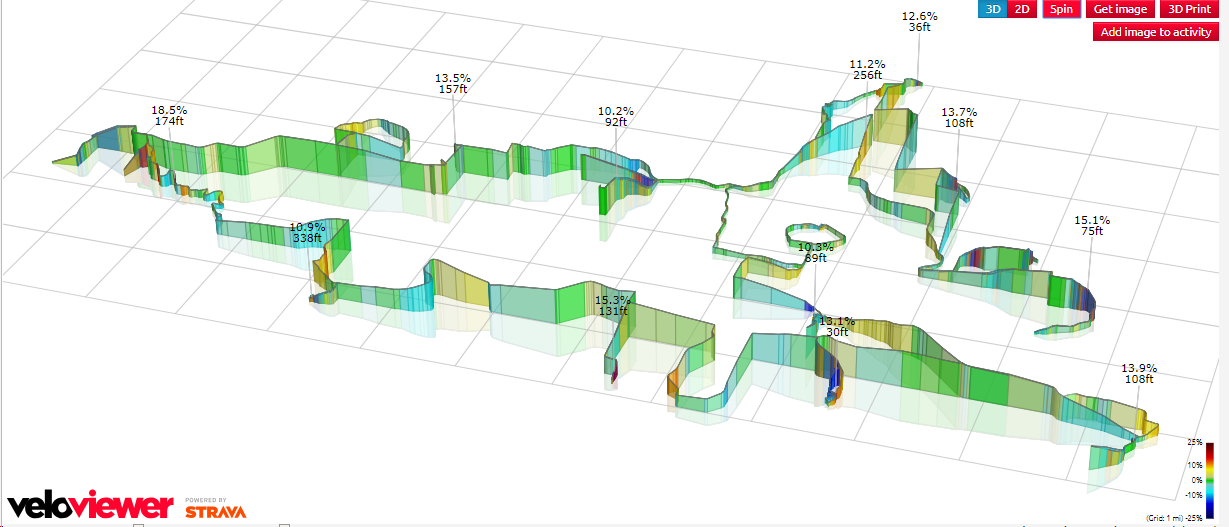